The source code of all applications is included in the JGameGrid distribution.
Space Invaders
is an arcade video game designed by Tomohiro Nishikado and released in 1978. It is considered one of the most successful arcade shooting games. The aim is to defeat waves of aliens with a laser cannon and earn as many points as possible. Though simple by today's standards, it was one of the forerunners of modern video gaming and helped expand the video game industry from a novelty to a global industry.
To keep the code simple and self-explaining, the application presented here does not implement all features of the full-fledged game. The spaceship can be moved horizontally with the left and right cursor keys. Each time the space bar is hit, a bomb is launched that may destroy an alien when hitting it. The aliens are moving left to right and approach the bottom. If the spaceship is hit by a alien, the game is over.
This implementation using JGameGrid clearly shows the advantage of using an object-oriented program design. A bomb is an instance of a class Bomb that extends the class Actor. Like in reality, once created, a bomb acts completely independently from other bombs and the rest of the program. By declaring the Bomb class we decouple the code to handle the bomb from the rest of the program. The act() method moves the bomb and detects if the bomb hits a alien. When this happens, an explosion actor is shown and both, the bomb and the alien, are destroyed. If the bomb misses all aliens and quits the battlefield, it is self-destroyed.
public class Bomb extends Actor
{
public void act()
{
move();
if (gameGrid.removeActorsAt(getLocation(), Alien.class) != 0)
{
Explosion explosion = new Explosion();
gameGrid.addActor(explosion, getLocation());
removeSelf();
}
if (getLocation().y < 5)
removeSelf();
}
}
Execute the program locally using WebStart.
Fifteen Puzzle
is the oldest type of sliding block puzzle, invented around 1880 by Noyes Chapman, a postmaster in the USA. It consists of a frame of numbered square tiles in random order with one tile missing. The object of the puzzle is to place the tiles in order by making sliding moves that use the empty space. Johnson and Story showed in 1879 that half of the starting positions for the puzzle are impossible to resolve, no matter how many moves are made. This is done by considering a function of the tile configuration that is invariant under any valid move.
[Ref. http://en.wikipedia.org/wiki/Fifteen_puzzle]
Because of the two-dimensional grid structure of the slide block puzzles, the implementation in JGameGrid is rather simple. For the Fifteen Puzzle we obviously use a 4x4 cell grid. Each block is an instance of a NumberBlock class that extends Actor and has its own GGMouseListener that handles the block when the left mouse button is pressed or released or the mouse is dragged.
When started, the 15 blocks are set at random cell positions. Bu only half of this starting situations are solvable. We change the initial condition until we get a solvable game (see Wikipedia reference how to do it).
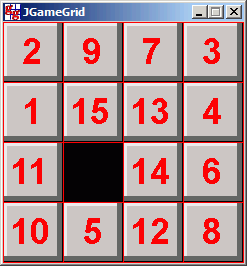
Execute the program locally using WebStart.
Lunar Lander
is an arcade game first released for the Atari computer in 1979. The object of the game is to pilot a lunar landing module to a safe touchdown on the moon. To pilot the lander, the player must counteract gravity by using the lander's aft thrusters to slow its descent. The player uses a proportional throttle to adjust the strength of the thrusters. Each action uses up the craft's limited fuel, and when fuel has run out, the lander stops responding to the player's actions.
[Ref. http://en.wikipedia.org/wiki/Lunar_Lander_%28arcade_game%29e]
Our implementation using JGameGrid is a simple example of a flight simulator that can be used to train pilots for a future space mission. The fuel throttle is manipulated by the cursor up and down keys. During the descent, relevant information is displayed in the title bar. In addition a voice informs about the remaining fuel mass. A background noise is heard with increasing volume when the throttle is opened. You also see the increasing burning gas outlet of the rocket motor.
This is a nice demonstration that combines physical knowledge, programming, graphics and sound. It's easily extended to allow horizontal movements.
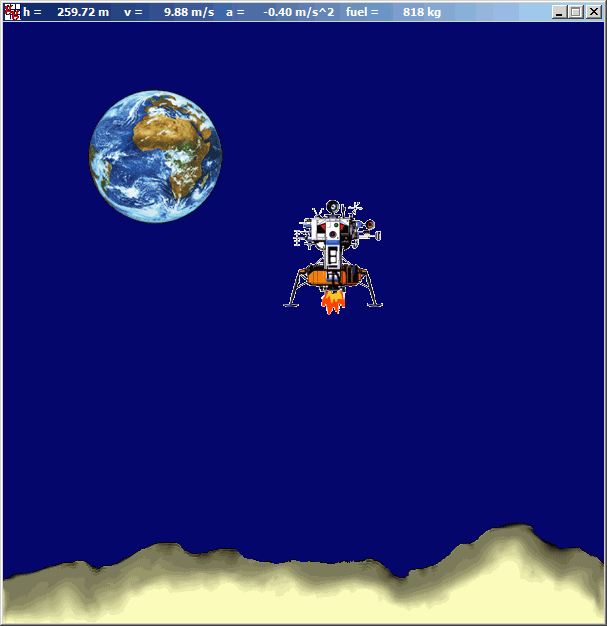
Execute the program locally using WebStart.
Three-body motion in Newtonian space
Given the masses, positions and velocities of three bodies in mutual gravitational attraction at a given starting time, the problem is to determine the motion of the bodies, e.g. the positions at any future time. This so-called three-body problem has attracted attentions of mathematicians and physicists over the past 300 years. The sun, the earth and the moon are a typical three-body system.
Despite the relative simplicity of the system, it is proven that the equations of motion of the system cannot be solved analytically in a general case. Therefore a computer simulation is needed.
Using the JGameGrid library the three bodies are considered to be instances of the class Body that extends Actor. In the body's act() method the total force exerted by the two other actors is determined using Newton's law of gravitation. Following Newton's second law of movement, the acceleration is the ratio of the force and the mass. The acceleration multiplied by the simulation period gives the linear approximation of the velocity change and finally the new position. It is convenient to use the class GGVector for all calculations.
public void act()
{
ArrayList<Actor> neighbours = gameGrid.getActors(CelestialBody.class);
neighbours.remove(this); // Remove self
GGVector totalForce = new GGVector();
for (Actor neighbour : neighbours)
{
CelestialBody body = (CelestialBody)neighbour;
GGVector r = body.getPosition().sub(position);
double rmag = r.magnitude();
GGVector force = r.mult(G * mass * body.getMass() / (rmag * rmag * rmag));
totalForce = totalForce.add(force);
}
acceleration = totalForce.mult(1 / mass);
velocity = velocity.add(acceleration.mult(timeFactor));
position = position.add(velocity.mult(timeFactor));
setLocation(toLocation(position));
}
The traces are drawn using JGameGrid's background graphics. This code is completely independent of the number of bodies, thus it's a snap to extend the program to more than 3 bodies. For the sake of simplicity the initial conditions are hard-coded. The demonstration shows that the system is instable and one of the bodies is ejected. Analyzing more deeply the movement reveals lot of material to be discussed in a physics course.
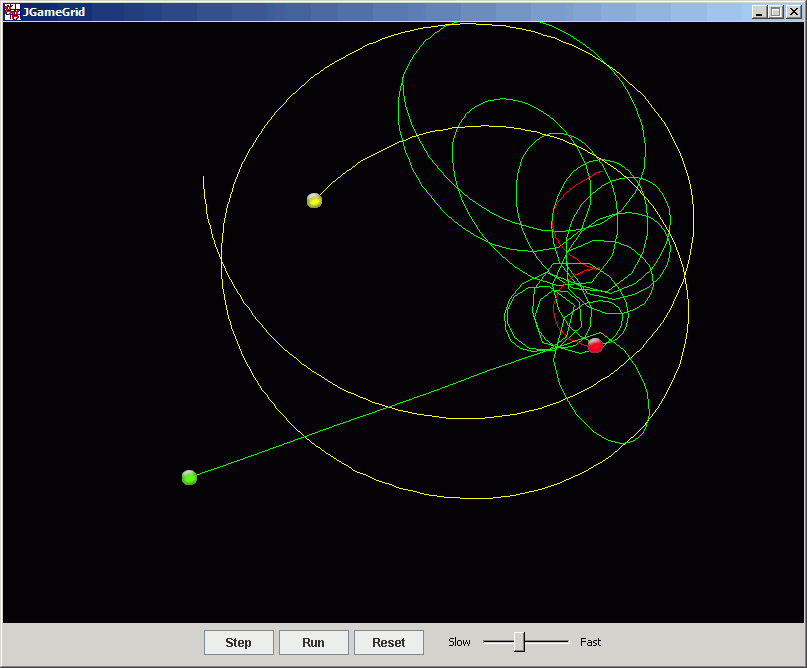
Execute the program locally using WebStart.
|