Utility
Package For Java
A programming language is almost useless if it is not accompanied by a high-performance library of routines, functions or classes. A library may be part of the language standard like in C++ or a language version like in Java. In Java the system library is called Application Programming Interface (API) and contains thousands of classes with tens of thousands of methods. Even so, there is necessity for more code pools for dedicated programming environments, and people all over the world develops their own programming libraries. Because they think that other people may have the same need, they often release their library to the public for free via the internet, ready to use (compiled) or even the source code. They generously give away some part of their intellectual propriety to the programming community expecting to contribute to the development of computing.
This contribution consists of a Java class library especially designed for the learning environment. It is fully admitted that children and young students are particularly "vision based": Visual inputs are very important for the learning process and it seems that images are better kept in long-term memory than other sensations. Therefore the easiest and most motivating way to introduce into programming is by using graphics. Most modern programming learning environments do it in this way. They may even use graphical programming languages where the code is represented by icons.
Since the 1970 when we started teaching programming on DEC minicomputers, we used graphics terminals and plotters for all students. When personal computers (IBM, Apple, DEC Professional) came available, graphics libraries were developed and extensively used in all programming courses. The programming languages changed from Basic to Pascal to C++ and finally to Java, but the concept of the graphics library remained the same: To supply a simple graphics window with either coordinate based or direction based graphics command. The latter was normally implemented like a LOGO turtle. With the better performance of the graphics system and the use of OOP, the turtle became an object with faculties and attributes. OOP-based turtle graphics became a wonderful vehicle to introduce OOP from the very beginning.
Therefore it was our first duty to develop a Java packages for coordinate graphics (ch.aplu.util) and turtle graphics (ch.aplu.turtle) when we started teaching Java in the late 1990. These packages have been continuously maintained and improved to keep them up-to-date.
Here two examples to show how simple it is to use the coordinate-based GPanel and the direction-based Turtle.
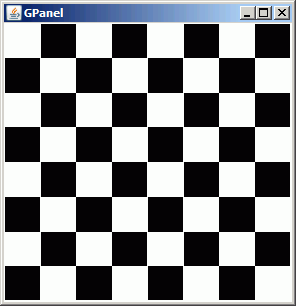 |
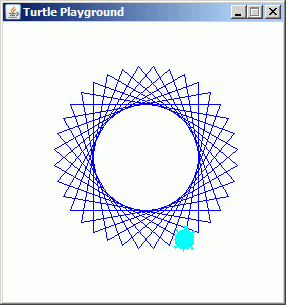 |
import ch.aplu.util.*;
class Chessboard extends GPanel
{
Chessboard()
{
window(0, 8, 0, 8);
for (int i = 0; i < 8; i++)
for (int j = 0; j < 8; j++)
if ((i + j) % 2 == 0)
fillRectangle(i, j, i + 1, j + 1);
}
public static void main(String[] args)
{
new Chessboard();
}
}
|
import ch.aplu.turtle.*;
class TurtleStar
{
public static void main(String[] args)
{
Turtle t = new Turtle();
for (int n = 1; n < 50; n++)
{
t.forward(100);
t.left(110);
}
}
}
|
The package ch.aplu.util contains some other useful library classes:
- Console window
- Precise timer
- Sound player (WAV and MP3)
- Input and modeless dialogs
For more information consult the following documentations:
JavaDoc for package ch.aplu.util
JavaDoc for package ch.aplu.turtle
|