The Fischertechnik robot kits
The Fischertechnik system is based on plastic building blocks that can be assembled to large constructions and equipped with motors, gears and numerous electronic components. Fischertechnik was founded by the German inventor Artur Fischer in 1964 and contributes to the technical education for hobbyists and students in educational institutions (general, technical and engineering schools). The robot production line consists of robot kits that models different kinds of real robots like robot rovers equipped with sensors and industrial robots with moving picker arms. These robots are controlled by a sophisticated and well-designed controller interface that drives motors, relays, lamps and reads analog and digital values. A program developed and running on a PC communicates with the controller interface via USB or a wireless communication channel in real time. With a special graphical programming environment (Robo Pro) a program can also be downloaded into the controller and run locally. In Java downloading of programs is not supported.
|
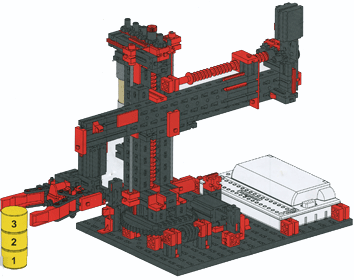 |
|
The Industry Robots II model |
How it works
The Java FtController
library uses a DLL umFish40.DLL developed by Ulrich Müller.
This DLL is based on the FtLib library distributed by fischertechnik.
Because umFish40.DLL conforms to the VC standards for exposing
its functions, the Java API Wrapper framework (JAW)
can be easily used, while JAW is based on the Java Native Interface (JNI).
The FtController library is devoted to Object Oriented Programming
(OOP) and the Java event model. Every effort is made
to fulfill this contract. In order to provide high performance, the native
program polls the controller state in a separate high priority native
thread (typically every 20 ms). State changes are reported to Java via
a large message queue (FIFO). This queue is polled by a Java thread that
generates events and invokes the registered callback methods. With this
mechanism there is little risk that an event is lost even if the system
is heavily loaded. Despite the underlying system is quite complicated
and uses different DLLs running quite a bit of C++ code, the Java interface
offered to the user is kept as simple as possible and may be applied with
only basic Java knowledge.
System requirements
- Windows XP or Vista
- Java JRE
(Version 5 or higher).
- Java IDE recommended (e.g. Netbeans, Eclipse).
- USB controller driver installed
Download USB controller driver. Driver will also be installed by installing the RoboPro programming language.
A simple demonstration
The following program uses a motor that turns a spindle with a impulse wheel. The wheel actuates a switch (step switch) connected to the digital input port I2. A second switch (end switch) is connected to input port I1. It is used to stop the motor at a predefined position (home position). When run, the motor turns 30 steps to the right. It then turns 40 steps to the left or until the end switch is pressed. The stop and step actions are reported by the MotorListener's callback methods.
// RobModeDemo.java
// Hardware setup:
// Motor at port M1, end (limit) switch at I1, step switch at I2
import ch.aplu.ftcontroller.*;
import ch.aplu.util.*;
public class RobModeDemo implements MotorListener, ExitListener
{
private FtController fc = new FtController();
public RobModeDemo()
{
Motor motor = fc.motor1;
motor.setSpeed(1);
fc.addMotorListener(this, motor);
Console c = Console.init();
c.addExitListener(this);
motor.right(30, true);
motor.left(40, true);
}
public void motorStopped(int motorNb, boolean isEnd)
{
System.out.print("Motor " + motorNb + " stopped");
if (isEnd)
System.out.println(" (end switch pressed)");
else
System.out.println(" (step switch counter reaches zero)");
}
public void stepChanged(int motorNb, int step)
{
System.out.println("# of steps: " + step);
}
public void notifyExit()
{
fc.exit();
}
public static void main(String[] args)
{
new RobModeDemo();
}
}
Execute RobModeDemo using WebStart.
If the execution fails, check the system requirements (see above)
Discussion: All real robot accessories like motors, lamps, counters, analog or digital ports, etc. are modeled by a corresponding Java object. When a FtController object is created, these objects are instantiated and are public parts of the FtController object. The parameterless constructor of FtController automatically tries to connect to the controller hardware. If it fails, an error message is displayed.
Due to the underlying event model, no polling is necessary to display the current number of steps. In a natural attitude, the halt of the motor is considered as an event and reported by the motorStopped() callback.
We display the System.out information in a convenient console window from the package ch.aplu.util.Console. Because we register an ExitListener, hitting the title bar's close button will call notifyExit() that releases all resources and terminates the application.
|