|
The source code of all examples is included in the JGameGrid distribution.
Ex12: Making Applets
For simple game applications and in a beginners course is it advisable to use the build-in window and navigation bar provided by the GameGrid class. But as we have seen in the very first lesson, JGameGrid offers the possibility to include its graphics pane into you own frame window using a GUI builder. The same technique is applied to integrate a game playground into an applet. If you are already somewhat accustomed to JGameGrid you will find it easy to create an applet. J just pay attention to the following guidelines:
-
In order to test your applet or to distribute the game as an application also, it is a good idea to design your program as applet and application at the same time. So it contains a main() method as entry point for your application.
-
Your main class must be derived from JApplet and the methods init(), start() and stop() should be overridden. These methods are called from the applet container that is controlled by the Web browser. Normally no constructor is used.
-
init() is used to create and initialize the class GameGrid. You must use the default constructor (parameterless) because the applet will be embedded into your Web page. Use the GameGrid initializer methods setNbHorzCells(), setNbVertCells(), setCellSize(), etc. instead of the constructor parameters. The navigation bar is not available for applets.
-
You add your GameGrid reference to the content pane of the JApplet by calling getContentPane.add().
-
In the start() method you start the simulation cycling by calling doRun(). There is no need for a separate thread because the simulation loop runs in its own internal thread.
-
The user will be grateful if you stop this thread when the Website is abandoned by calling stopGameThread().
-
For the standalone version you declare an initializer method initStandalone() where you put the code that creates the JFrame in the usual manner. Only one thing is really important: All graphics operations of JGameGrid have to be postponed until the window is completely setup. This is not the case until pack() has been called. Normally the simulation cycling should not be started before, because the act() methods usually perform graphical operations.
- It is somewhat a trick, but it simplifies the code to add the JApplet content pane to the JFrame content pane by calling f.setContentPane(getContentPane()).
|
In our demonstration a lot of randomly generated spiders produces shaky lines of silk. As usual we first design the actor class Spider. It is easy to show the silk by using the graphics line drawing of the GGBackground class. To get the background reference in an Actor class we use the public GameGrid reference gameGrid. As the spiders have some troubles in keeping a straight line, we give them some randomized direction. After they leave the bottom border we release the memory by calling removeSelf().
import ch.aplu.jgamegrid.*;
public class Spider extends Actor
{
private int xOld, yOld;
public Spider()
{
super(true, "sprites/spider.gif");
}
public void act()
{
move();
int x = getX();
int y = getY();
gameGrid.getBg().drawLine(xOld, yOld, x, y);
xOld = x;
yOld = y;
setDirection(70 + 40 * Math.random());
if (getY() > 220)
removeSelf();
}
}
|
Now we create the main program following closely our above-mentioned applet recipe. There is few to explain furthermore. We use the act() method to generate the spiders at random x-positions. To limit somewhat the production rate, we use getNbCycles() to make a new spider every tens cycle.
import ch.aplu.jgamegrid.*;
import javax.swing.*;
import java.awt.*;
public class Ex12 extends JApplet implements GGActListener
{
private GameGrid gg;
public void init()
{
gg = new GameGrid();
gg.setNbHorzCells(400);
gg.setNbVertCells(200);
gg.setCellSize(1);
gg.setSimulationPeriod(100);
gg.setBgColor(new Color(182, 220, 234));
gg.addActListener(this);
getContentPane().add(gg);
}
public void start()
{
gg.doRun();
}
public void stop()
{
gg.stopGameThread();
}
public void act()
{
if (gg.getNbCycles() % 10 == 0)
{
int x = 5 + (int)(390 * Math.random());
gg.addActor(new Spider(),
new Location(x, -20), 90);
}
}
private void initStandalone()
{
init();
JFrame f = new JFrame();
f.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
f.setContentPane(getContentPane());
f.pack(); // Must be called before start()
start();
f.setVisible(true);
}
public static void main(String[] args)
{
EventQueue.invokeLater(new Runnable()
{
public void run()
{
new Ex12().initStandalone();
}
});
}
} |
Execute the program locally using WebStart.
To use the program as an applet, all compiled classes and the sprites subdirectory containing spider.gif are packed into a JAR file Ex12.jar. For security reasons it is necessary to add a MANIFEST.MF that contains the following entries:
Manifest-Version: 1.0
Ant-Version: Apache Ant 1.8.4
Created-By: 1.6.0_01 (Sun Microsystems Inc.)
Application-Name: *
Permissions: all-permissions
Main-Class: Ex12
Codebase: *
(the first three lines may have other versions)
The JAR file has to be signed using the JarSigner (see Internet resources to learn how to sign a JAR archive). The same signature must be used to sign the library JAR JGameGrid.jar that also must contain a MANIFEST.MF.
(Download Ex12.jar for examination)
For the purpose of a simple demonstration we compose a rudimentary HTML file ex12.html and copy it together with JGameGrid.jar, Ex12.jar into the public space of a Web server.
<html>
<body>
<div align="center">
<h1>Ex12 - JGameGrid Applet</h1>
</div>
<div align="center">
<table width="401" border="1" cellspacing="0" cellpadding="10">
<tr>
<td width="401">
<applet code="Ex12.class" archive="JGameGrid.jar, Ex12.jar"
width="401" height="201">
</applet>
</tr>
</table>
</div>
</body>
</html> |
Click
here to see the result.
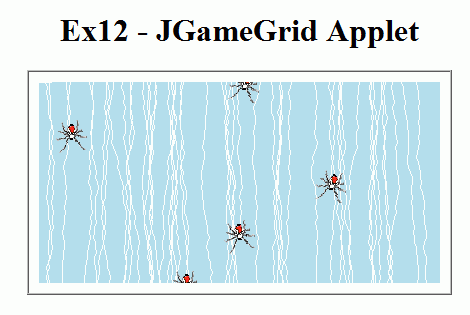
| |