|
The source code of all examples is included in the JGameGrid distribution.
Playing Sound
In many game applications sound not only provides more fun and action, but adds a new dimension for user interaction. The player gets informed about special game situations by an additional channel of perception which may be an important part of the game design. With JGameGrid you can simply play a short sound clip by calling the playSound() method, but you can also manage longer sound resources by using the SoundPlayer class from the ch.aplu.util package. SoundPlayer implements the common commands like play, pause, resume, stop and volume changes.
The audio file may be packed in the application JAR or retrieved from the local or remote file system. Standard support is for the WAV audio format, but with some additional library jars, sound from MP3 audio files can be played too.
There are a few sound clips integrated in the JGameGrid jar distribution that are accessible with GGSound constants. You can hear some of them in the first example by hitting a keyboard key.
import ch.aplu.jgamegrid.*;
import java.awt.event.KeyEvent;
public class Ex28 extends GameGrid implements GGKeyListener
{
private GGTextField tf1;
private GGTextField tf2;
public Ex28()
{
super(300, 60, 1, false);
getBg().clear(WHITE);
addKeyListener(this);
tf1 = new GGTextField(this, "System Sound Demo. Active keys: A to D",
new Location(10, 10), false);
tf2 = new GGTextField(this, "Currently playing: DUMMY",
new Location(10, 30), false);
tf1.show();
tf2.show();
playSound(GGSound.DUMMY); // Speeds-up start of first sound clip
show();
}
public boolean keyPressed(KeyEvent evt)
{
switch (evt.getKeyCode())
{
case KeyEvent.VK_A:
playSound(GGSound.BOING);
showInfo("BOING");
break;
case KeyEvent.VK_B:
playSound(GGSound.FADE);
showInfo("FADE");
break;
case KeyEvent.VK_C:
playSound(GGSound.NOTIFY);
showInfo("NOTIFY");
break;
case KeyEvent.VK_D:
playSound(GGSound.EXPLODE);
showInfo("EXPLODE");
break;
}
refresh();
return true;
}
public boolean keyReleased(KeyEvent evt)
{
return false;
}
private void showInfo(String text)
{
tf2.setText("Currently playing: " + text);
}
public static void main(String[] args)
{
new Ex28();
}
} |
Execute the program locally using WebStart.
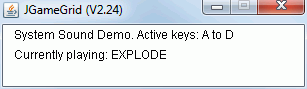
To play your own sound clips, you use the SoundPlayer or SoundPlayerExt class from the ch.aplu.util package (included in the JGameGrid.jar distribution). To play WAV files, put the file in a subdirectory, e.g. "wav", of the project source directory and use the SoundPlayer constructor that takes the relative path to the WAV resource. When your application is packed into a JAR file, the wav subdirectory containing the sound file must be included in the JAR (the SoundPlayer constructor will automatically load it from the JAR). If the sound clip is short, just call the play() method. Keep in mind that play() returns immediately without waiting for the clip to finish. SoundPlayer provides methods for controlling the player in details and a SoundPlayerListener may be registered to report the state of the sound player. This is very useful if you need to wait until the clips is at its end. We demonstrate some of these features in the next example.
import ch.aplu.util.*;
import ch.aplu.jgamegrid.*;
import java.awt.event.KeyEvent;
public class Ex28a extends GameGrid
implements GGKeyListener, SoundPlayerListener
{
private SoundPlayer player;
private int volume = 1000;
private GGTextField tf1;
private GGTextField tf2;
private GGTextField tf3;
public Ex28a()
{
super(400, 60, 1, false);
getBg().clear(WHITE);
addKeyListener(this);
tf1 = new GGTextField(this,
"Keys: G (Go), S (Stop), P (Pause). UP (Vol+), DOWN (Vol-)",
new Location(10, 10), false);
tf2 = new GGTextField(this, "", new Location(10, 30), false);
tf3 = new GGTextField(this, "", new Location(10, 50), false);
tf1.show();
tf2.show();
tf3.show();
show();
// Use sound ressource from application JAR
player = new SoundPlayer(this, "wav/kid.wav");
player.addSoundPlayerListener(this);
player.setVolume(volume);
}
public boolean keyPressed(KeyEvent evt)
{
switch (evt.getKeyCode())
{
case KeyEvent.VK_G:
player.play();
tf2.setText("PLAY");
break;
case KeyEvent.VK_S:
player.stop();
tf2.setText("STOP");
break;
case KeyEvent.VK_P:
player.pause();
tf2.setText("PAUSE");
break;
case KeyEvent.VK_DOWN:
if (volume != 500)
volume -= 10;
player.setVolume(volume);
tf2.setText("Volume: " + volume);
break;
case KeyEvent.VK_UP:
if (volume != 1000)
volume += 10;
player.setVolume(volume);
tf2.setText("Volume: " + volume);
break;
}
refresh();
return true;
}
public boolean keyReleased(KeyEvent evt)
{
return false;
}
public void notifySoundPlayerStateChange(int reason, int mixerIndex)
{
switch (reason)
{
case 0:
tf3.setText("Playing from start");
break;
case 1:
tf3.setText("Resume playing after pause");
break;
case 2:
tf3.setText("Pausing");
break;
case 3:
tf3.setText("Stopping");
break;
case 4:
tf3.setText("End of sound resource");
break;
}
refresh();
}
public static void main(String[] args)
{
new Ex28a();
}
} |
Execute the program locally using WebStart.
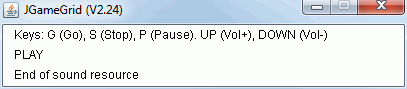
Unfortunately the standard Java sound API has no provision to play audio files in MP3 format, but SoundPlayerExt will open the way. Just replace SoundPlayer in Ex28a by SoundPlayerExt and add the following JAR resources (or later versions) to the runtime classpath:
- jl1.0.1.jar
- mp3spi1.9.4.jar
- tritonus_share.jar
(You may download them freely from http://www.sourceforge.net or a redistribution from http://www.aplu.ch/mp3support)
| |