A Java Package
To Learn Programming Using Android Smartphones/Tablets
Back To The Roots: Turtle Graphics
There is no need to explain what Turtle Graphics is nor to emphasize its importance in computer programming methodology. Since the invention of Logo in 1967, turtle libraries became available for many programming languages. In some teaching environments Turtle Graphics is even the language itself. It is a success story until now and Turtle Graphics is used to teach programming fundamentals from first year school children to university students. The natural addition of OOP concepts where turtles are objects that may interact together in a common playground, makes it easy to demonstrate modern programming concepts. Porting Turtle Graphics to smartphones and tablets adds another touch of modernity that motivates students to learn programming.
One major difference between turtle geometry and coordinate geometry rests on the notation of the intrinsic properties of geometric figures. An intrinsic property is one which depends only on the figure in question, not on the figure's relation to a frame of reference. The fact that a rectangle has four equal angles is intrinsic to the rectangle. But the fact that a particular rectangle has two vertical sides is extrinsic, for an external reference frame is required to determine which direction is vertical. (From the very recommendable book by Abelson and diSessa, Turtle Geometry, MIT Press 1986)
As you see in the following examples, JTurtleLib is a lightweight Turtle Graphics implementation for Android that lets you demonstrate fundamental programming paradigms like procedural/functional programming, object-oriented design and event-driven architecture with just a few lines of code.
1 The procedural paradigm
There is a method (procedure) main(), where the actions (statements) are noted line-per-line. This defines the order the actions are executed when the program is started. To tell the the global turtle what to do, you write actions (turtle commands) like
"move forward 100 steps" : forward(100) or fd(100)
"turn to the right 160 degrees" : right(160) or rt(160)
package turtle.tut01;
import turtle.*;
public class Tut01 extends Playground
{
public void main()
{
st();
while (true)
{
fd(100);
rt(160);
}
}
|
|
This short program creates a fully functional Android app that can be installed on any smartphone or tablet. The coordinate system and the size of the turtle are automatically adapted to the current device.
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
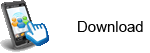 |
source (Tut01.zip). |
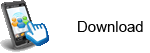 |
Android app for installation on a smartphone or emulator. |
The playground is the region (and the programming unity) where the turtles live. A turtle called "global turtle" is created by the system, but still invisible when main starts to execute. To show the global turtle, you call showTurtle() or st() at the beginning of main.
Program dissection line-per-line:
There is a somewhat boring program header that prepares the program environment:
package turtle.tut01; << Creates an identification for our App on the smartphone/tablet
import turtle.*; << Gives us access to the JTurtle library
public << Makes the class "visible" to the Android operating system, so it can be executed
class Tut01 << Defines a programming unit called a "class"
extends Playground << Creates a playground and gives us access to all its features
Here, the Android operating system starts the program execution:
publc void main() << Is the "entry point" of the program, 'void' says that when main terminates, it returns "nothing"
Here come the real program statements, executed in sequence at run-time:
st(); << Shows the hidden global turtle
while (true)<< Repeats the following block indefinitely
(Not a statement per se, so do not terminate line with a semicolon!)
fd(100); << Moves the turtle 100 steps in forward direction
rt(160); << Turns the turtle 160 degrees to the right
2 The object-oriented (OO) paradigm
In a OO program, a turtle is represented by an instance of the Turtle class. This view is very natural, because real turtle animals are also member of a biological class. To give us a concrete feeling, we use a female name for our turtle. To tell a turtle what to do, we use its name (like shouting) and the command (called method) separated by a dot. As you see, the turtle movements are animated, so you can better follow the program execution. The turtle speed may be set individually for each turtle and if you set it to MAXSPEED, the movements are fast and unanimated.
package turtle.tut02;
import turtle.*;
public class Tut02 extends Playground
{
public void main()
{
Turtle lisa = new Turtle();
while (true)
{
lisa.fd(100);
lisa.rt(160);
}
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
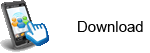 |
source (Tut02.zip). |
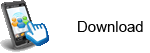 |
Android app for installation on a smartphone or emulator. |
Program dissection of modified lines:
Turtle lisa = new Turtle(); << Creates a new turtle object named 'lisa' and shows it in home position
lisa.fd(100); << Sends the message 'go forward 100 steps' to lisa (in Java called 'method call')
lisa.rt(160); << Sends the message 'turn right 160 degrees' to lisa
Because lisa is a turtle "object", why not create other turtle objects like peter and laura?
Turtle peter = new Turtle(BLUE); << Creates a blue colored turtle named 'peter'
Turtle laura = new Turtle(RED); << Creates a red colored turtle named 'laura'
Let move peter and laura in harmony with lisa by adding some commands (forward (fd), back (bk), left (lt) or right (rt) separated by a dot). If a turtle meets other anmials at the same location, the turtle slides over them without harm. Try it!
3 The event-driven paradigm
Like in common life an event happens when something special occurs. Unlike in standard programs, where one thing happens after the other in well-defined order, event-driven programs have named code blocks (called callbacks or notification methods) that just sit there waiting to be invoked when a certain event happens. Think about your smartphone doing nothing, just waiting for a incoming phone call. When this event happens it wakes up and the dormant code is executed that alarms you as predefined by the user settings. Another typical event on smartphone if fired when your finger taps the touch screen. Of course the current tap location (as x-y coordinates) is passed to a notification method.
All what you need to know is how the notification methods are named. In JTurtleLib, the predefined method name for the event that is triggered when your finger touches the screen is called playgroundPressed. Two decimal numbers x and y are passed to the method. They represent the location of the finger tap in the turtle playground coordinate system (x: -200 to 200, left to right; y: -200 to 200 bottom to top). The coordinate system is independent of the device screen resolution, so you don't have to worry about screen pixels.
package turtle.tut03;
import turtle.*;
public class Tut03 extends Playground
{
public void playgroundPressed(double x, double y)
{
Turtle lisa = new Turtle(x, y);
while (true)
{
lisa.fd(100);
lisa.rt(160);
}
}
}
|
|
Open source in Online-Compiler. (You need the latest version of JRE.)
Create QR code to download Android app to your smartphone.
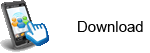 |
source (Tut03.zip). |
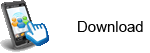 |
Android app for installation on a smartphone or emulator. |
Program dissection of modified lines:
public void playgroundPressed(double x, double y) << Event notification method
(must be 'public' because the operating system invokes it)
Turtle lisa = new Turtle(x, y); << Creates a turtle at given position
When you tap the screen, a new turtle named 'lisa' is created at the touch position and lisa performs the drawing of the star. All these turtle instances lives in harmony together and overlap when necessary. There is no conflict between each instance. It is like in real life, where also more than one girl named Lisa exist and make a drawing at the same time ("in parallel").
This program gives you a real feeling of events and multi-threading. It is hard to find a better example of modern program design, where code is "hanging around" doing nothing until some event happens. While the code is executing, another event may be fired and the same code runs in parallel.
The JTurtleLib class design:
JTurtleLib is based on JDroidLib, a user friendly game-oriented library for Android ported from the Java SE JGameGrid framework. The Playground class is extended from the GameGrid class that extends Activity, but all public methods of the base class are declared protected, so they do not show up in the class documentation. This special class design is necessary because the Android OS requires a subclass of Activity to start the application. Playground exposes (but does not document) all methods of the GameGrid class as documented in the JDroidLib reference. Turtles look like JDroidLib actors, but the Actor class is hidden by putting the class Turtle in a has-a relation with the Actor class. To gain access to the underlying Actor, use Turtle.getActor(). |