Cloud Computing
In many IoT applications, the storage of sensor values in the cloud is of particular interest, since this data can then be retrieved from anywhere via the Internet.
We use the ThingSpeak cloud server, which is available free of charge for small data volumes. In addition, this cloud service offers Mathworks (distribution from MathLab), with which data can be easily displayed graphically as part of an Web site.
To use the services, you need to create a personal account with ThingSpeak (www.thingspeak.com). Data is organized in tables called channels. First you define a new channel by selecting a name (e.g. Sensor Data) and a field descriptor (column name, e.g. Temperature (degC)). Under Channel Sharing Setting, select Share channel with everyone. Under API Keys write down the Write and Read API Keys.
Unter API Request you get the information how to enter a new value (Update a Channel Feed) using a HTTP or HTTPS GET request, e.g.
https://api.thingspeak.com/update?api_key=Y03F0Y1IPUKP6XYZ&field1=25
You may copy this line to the browser URL and see the result under My Channels.
The following program connects to a access point and sends a new temperature value every 20 s (for a free account the maximum data rate is 1 sample every 15 s). The temperature is also displayed digit by digit on micro:bit's display and written into the console. The HTTP response received from the cloud server is also shown in the console. It is the total number of the channel values.
# ThingSpeakTemp.py
from microbit import *
from linkup import *
import sht
key = "TJH7REA0WQD0QXYZ"
print("Sending AP connection command")
connectAP("myssid", "mypassword")
while True:
temp, humi = sht.getValues()
print("Sending new temperature:", temp)
v = "%3.1f" % temp
for c in v:
display.show(c)
sleep(500)
display.clear()
sleep(500)
url = "http://api.thingspeak.com/update?api_key=" + key + "&field1=" + str(temp)
response = httpGet(url)
print("Response:", response)
sleep(20000)
You can retrieve the channel data of a public channel with a HTTP GET request, e.g. the last 100 entries with
url = https://api.thingspeak.com/channels/" + channelID + "/feeds.json?results=100"
where channelID is taken from your ThinkSpeak account. The data is json-formattet string that is similar to a Python dictionary. By using the eval() function you convert it to a dictionary with no effort.
Using TigerJython's graphics window GPanel, it is easy to visualize the last 100 entries.
# ThingSpeakTempDisplay.py
import urllib2
from gpanel import *
makeGPanel(-10, 110, -5, 55)
drawGrid(0, 100, 0, 50)
lineWidth(2)
x = 0
channelID = "765177"
url = "https://api.thingspeak.com/channels/" \
+ channelID + "/feeds.json?results=100"
data = urllib2.urlopen(url).read()
dataDict = eval(data)
print dataDict
feeds = dataDict['feeds']
for feed in feeds:
a = feed['field1']
y = float(a)
if x == 0:
pos(x, y)
else:
draw(x, y)
x += 1
|
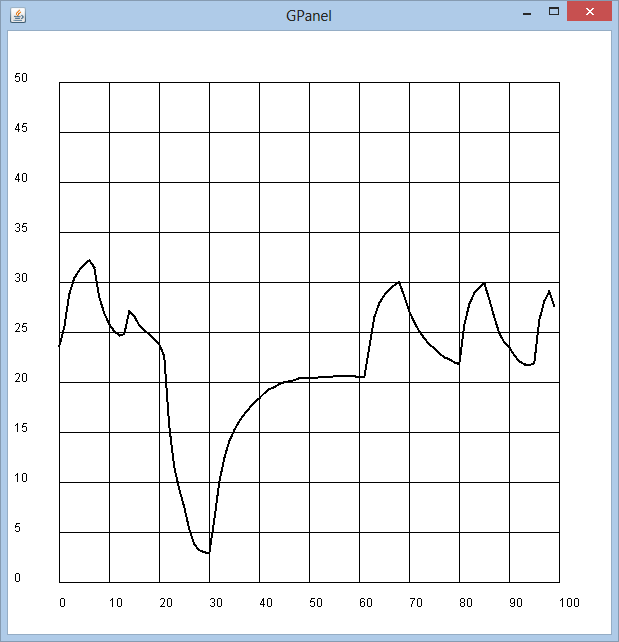 |
In the next program, data is saved line per line in a text file temp.txt where x and y are separated by a semicolon. Then the file is read with a spreadsheet and data displayed as graphics.
# ThingSpeakTempSave.py
import urllib2
from gpanel import *
channelID = "765177"
url = "https://api.thingspeak.com/channels/" \
+ channelID + "/feeds.json?results=100"
data = urllib2.urlopen(url).read()
dataDict = eval(data)
feeds = dataDict['feeds']
x = 0
with open("temp.txt", "w+") as f:
for feed in feeds:
y = float(feed['field1'])
f.write("%d;%.2f\n" %(x, y))
x += 1
print "done" |
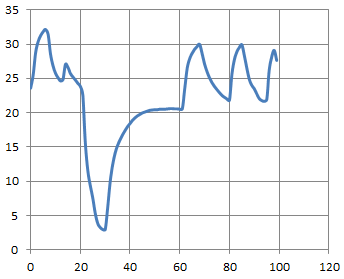 |
|