Since the Android OS uses Java as programming language, it was straightforward to port the Java SE based library TCPCom to Android. The API is exactly the same as for Java SE and the only difference is the fact that in verbose mode the information is written to a Android Log Console with the default tag "ch.aplu.android" (and not to System.out).
When the JDroidLib framework is used, the additional library TcpDroid.jar is simply added in the ProjectBuilder dialog, e.g.
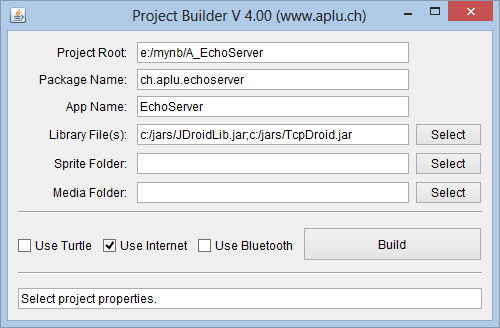
An echo server using a console window looks like this:
package ch.aplu.echoserver;
import ch.aplu.android.*;
import ch.aplu.tcpcom.*;
public class EchoServer extends GameGrid implements TCPServerListener
{
private TCPServer server;
private GGConsole c;
public void main()
{
int port = 5000;
c = GGConsole.init();
c.println("Echo server started");
server = new TCPServer(port);
server.addTCPServerListener(this);
}
public void onStateChanged(String state, String msg)
{
if (state.equals(TCPServer.LISTENING))
c.println("Waiting for a client...");
else if (state.equals(TCPServer.MESSAGE))
{
c.println("Received msg: " + msg + " - echoing it...");
server.sendMessage(msg);
}
}
}
|
Download Android app for installation on a smartphone or emulator.
Create QR code to download Android app to your smartphone.
It may be accessed from a client running a TCPCom client on any PC or another Android device like this:
package ch.aplu.echoclient;
import ch.aplu.android.*;
import ch.aplu.tcpcom.*;
public class EchoClient extends GameGrid implements TCPClientListener
{
private GGConsole c;
public void main()
{
String host = askIPAddress();
int port = 5000;
c = GGConsole.init();
c.println("Hello. Trying to connect " + host);
TCPClient client = new TCPClient(host, port);
boolean success = client.connect(10); // Timeout 10 s
if (!success)
{
c.println("Connection failed");
return;
}
client.addTCPClientListener(this);
c.println("Connection established. Sending now...");
for (int i = 0; i < 100; i++)
{
client.sendMessage("" + i);
delay(100); // slow down
}
c.println("Program terminated");
client.disconnect();
}
public void onStateChanged(String state, String msg)
{
if (state.equals(TCPClient.MESSAGE))
c.println("Got: " + msg);
else if (state.equals(TCPClient.DISCONNECTED))
c.println("Disconnected");
}
private String askIPAddress()
{
GGPreferences prefs = new GGPreferences(this);
String oldName = prefs.retrieveString("IPAddress");
String newName = null;
while (newName == null || newName.equals(""))
{
newName = GGInputDialog.show(this, "EV3", "Enter IP Address",
oldName == null ? "10.0.1.1" : oldName);
}
prefs.storeString("IPAddress", newName);
return newName;
}
}
|
Download Android app for installation on a smartphone or emulator.
Create QR code to download Android app to your smartphone.
Of course TcpDroid.jar may be added as external library to any other Android application builder.
|