Examples for the very first Java lesson using JavaOC:
Do some simple calculations:
|
// MyFirst.java
class MyFirst
{
MyFirst()
{
int items = 1234;
double price = 2.55;
double toPay = items * price;
System.out.print(toPay);
}
}
|
|
For a quick demonstration without an IDE you compile from a command shell with javac MyFirst.java and run the program with javaoc MyFirst. You may use constructors with primitive or string type parameters:
|
// MyFirstP.java
class MyFirstP
{
MyFirstP(int items, double price)
{
double toPay = items * price;
System.out.print(toPay);
}
} |
|
and pass the values as command line arguments, e.g.
javaoc MyFirstP 1234 2.5 .
You better use simple helper classes like ch.aplu.util.Console (see www.aplu.ch/java):
|
// MyFirstC.java
import ch.aplu.util.*;
class MyFirstC extends Console
{
MyFirstC()
{
print("Number of items: ");
int items = readInt();
print("Unit price: ");
double price = readDouble();
double toPay = items * price;
print("Amount to pay: " + toPay);
}
} |
|
which gives you a full-blown GUI application:
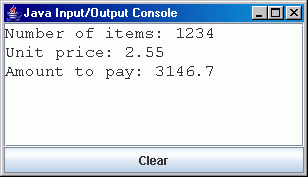
To let your students grow up in the OOP-world from the very first hour, use Logo-like turtles with classes ch.aplu.turtle.* (see www.aplu.ch/java):
|
// JagTurtle.java
import ch.aplu.turtle.*;
class JagTurtle extends Turtle
{
JagTurtle()
{
for (int i = 0; i < 4; i++)
{
jag();
right(60);
}
fill(-10, 10);
}
void jag()
{
forward(100);
left(150);
forward(100);
}
}
|
|
which declares a JagTurtle who "is-a" Turtle with a new "jag"-facility and make it draw a star:
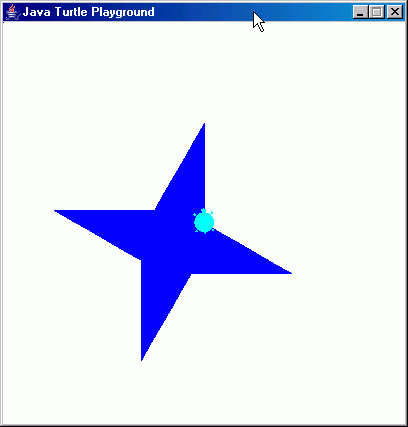
|