|
The source code of all examples is included in the JGameGrid distribution.
Ex17: Dynamic Sprite Images
Normally actor's sprite images are loaded from disk files in gif, jpg or png format. In some applications it may be necessary be create or transform the image at run-time by a program code. This approach is very flexible because static disk images can still be part of the actor image, but differently transformed using any of the many Java image processing classes. For dynamic sprite images, the actor constructor takes one or several references of BufferedImage instead of file paths. Actors with multiple sprites from any number of BufferedImage references are supported. Like with static sprites, buffered images passed to the Actor's constructor are hold in an internal sprite buffer and are automatically rotated when a rotatable actor is declared.
In the following example the sprite images of an actor represent a sine wave in forty successive positions. It would be very laborious to create all the pictures statically using a image editor. To construct the graphics, an instance of BufferedImage is created and the Graphics2D context extracted. Now any of the Graphics2D methods can be applied. Finally the buffered images are feed into the Actor constructor. Because createImages() that uses waveImage() is executed before the constructor is invoked, we must declare these methods static.
The actor's act() just cycles through all sprite images.
import java.awt.*;
import java.awt.image.BufferedImage;
import ch.aplu.jgamegrid.*;
public class WaveActor extends Actor
{
private static int nb = 40; // Number of sprites
public WaveActor(int width, int height)
{
super(createImages(width, height));
}
public void act()
{
showNextSprite();
}
private static BufferedImage[] createImages(int width, int height)
{
BufferedImage[] bis = new BufferedImage[nb];
for (int i = 0; i < nb; i++)
bis[i] = waveImage(width, height, width / nb * i);
return bis;
}
private static BufferedImage waveImage(int width, int height, int d)
{
BufferedImage bi = new BufferedImage(width, height, Transparency.BITMASK);
Graphics2D g2D = bi.createGraphics();
// Set background color
g2D.setColor(Color.blue);
g2D.fillRect(0, 0, width - 1, height - 1);
// Set line color
g2D.setColor(Color.white);
// Draw wave
double k = 2 * Math.PI / width;
double xOld = 0;
double yOld = 0;
double y;
double a = 0.4 * height;
for (int x = 0; x <= width; x += 1)
{
y = a * Math.sin(k * (x - d));
if (x == 0)
{
xOld = 0;
yOld = y;
}
else
{
Point ptOld = toPix(xOld, yOld, height);
Point pt = toPix(x, y, height);
g2D.drawLine(ptOld.x, ptOld.y, pt.x, pt.y);
xOld = x;
yOld = y;
}
}
return bi;
}
private static Point toPix(double x, double y, int height)
{
return new Point((int)x, height / 2 - (int)y);
}
} |
The application program creates the WaveActor instance and adds it to the GameGrid.
import ch.aplu.jgamegrid.*;
import java.awt.*;
public class Ex17 extends GameGrid
{
public Ex17()
{
super(600, 200, 1, false);
setSimulationPeriod(20);
setBgColor(Color.white);
addActor(new WaveActor(400, 100), new Location(300, 100));
show();
doRun();
}
public static void main(String[] args)
{
new Ex17();
}
} |
Execute the program locally using WebStart.
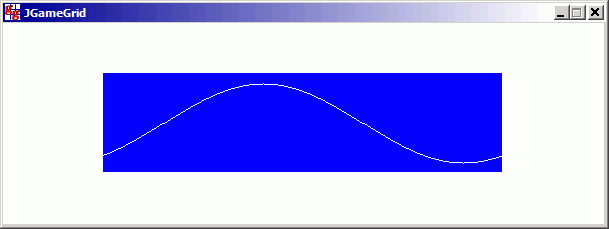
| |