Infrared Remote Control (Autonomous mode)
Purpose: Remote control with infrared controllers is widely used in everyday life. The EV3 infrared sensor may be used in Remote Control Mode as receiver of IR commands transmitted by the EV3 Infrared Transmitter.
The EV3 infrared sensor is set in remote mode when a IRRemoteSensor instance is created. The current button or the button combination is detected by calling the getCommand() method that returns an integer code between 0 and 11. A more user friendly enum is used to denominate the commands. It is up to you to define the robot's action performed by each remote command.
The motors are plugged into port A and B, the EV3 infrared sensor into port S4. The remote control unit supports four channels 1..4 selected by the red slide switch. The center button is a toggle button that turns on the infrared beacon. Do not forget to turn it off to save battery life.
import ch.aplu.ev3.*;
import lejos.hardware.lcd.LCD;
class RemoteIRControl
{
public enum Command
{
Nothing, TopL, BottomL, TopR,
BottomR, TopL_TopR, TopL_BottomR,
BottomL_TopR, BottomL_BottomR,
Center, BottomL_TopL, TopR_BottomR
}
private int channel = 1;
RemoteIRControl()
{
LegoRobot robot = new LegoRobot();
Gear gear = new Gear();
robot.addPart(gear);
IRRemoteSensor irs = new IRRemoteSensor(SensorPort.S4);
robot.addPart(irs);
LCD.drawString("IR Control Ready", 0, 1);
robot.playTone(600, 100);
while (!Tools.isEscapePressed())
{
int cmd = irs.getCommand(channel);
Command command = Command.values()[cmd];
switch (command)
{
case Center:
gear.stop();
break;
case TopL:
gear.leftArc(0.2);
break;
case TopR:
gear.rightArc(0.2);
break;
case TopL_TopR:
gear.forward();
break;
case BottomL:
gear.leftArc(-0.2);
break;
case BottomR:
gear.rightArc(-0.2);
break;
case BottomL_BottomR:
gear.backward();
break;
}
}
robot.exit();
}
public static void main(String[] args)
{
new RemoteIRControl();
}
}
Compilation/download using the OnlineEditor of PHBern (Bern University of Teacher Education)
|
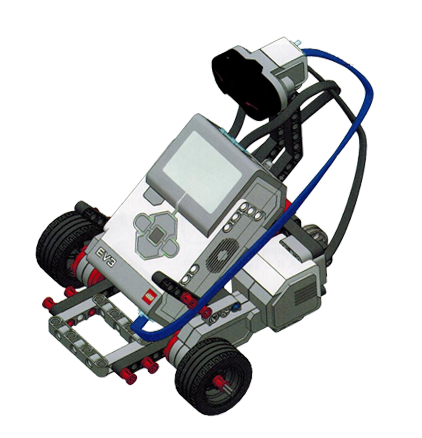 |
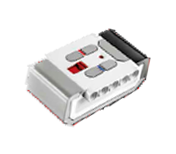 |
(Image from Valk, The Lego Mindstorms EV3 Discovery Book)
|
|