Border Follower (Autonomous Mode)
Purpose: The EV3 rover (standard model with two motors) equipped with a color or light sensor that distinguishes between a white and a black floor advances along a white-to-black border. The rover moves on a wiggly line and changes from a left to a right curve when the sensor 'sees' the black offshore region. |
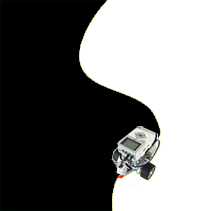 |
First you should check the light levels when the sensor detects the white or back floor.
import ch.aplu.ev3.*;
public class BorderFollower
{
public BorderFollower()
{
LegoRobot robot = new LegoRobot();
LightSensor ls = new LightSensor(SensorPort.S1);
robot.addPart(ls);
Gear gear = new Gear();
gear.setSpeed(20);
robot.addPart(gear);
while (!Tools.isEscapeHit())
{
if (ls.getValue() < 500)
gear.leftArc(0.2);
else
gear.rightArc(0.2);
}
robot.exit();
}
public static void main(String[] args)
{
new BorderFollower();
}
}
Compilation/download using the OnlineEditor of PHBern (Bern University of Teacher Education)
Border Follower (Direct Mode)
Purpose: Same as above, but in Direct Mode.
import ch.aplu.ev3.*;
public class BorderFollowerD
{
public BorderFollowerD()
{
LegoRobot robot = new LegoRobot();
LightSensor ls = new LightSensor(SensorPort.S1);
robot.addPart(ls);
Gear gear = new Gear();
gear.setSpeed(20);
robot.addPart(gear);
while (true)
{
if (ls.getValue() < 500)
gear.leftArc(0.2);
else
gear.rightArc(0.2);
}
}
public static void main(String[] args)
{
new BorderFollowerD();
}
}
Execute the program locally using WebStart.
Border Follower (Simulation Mode)
Purpose: Same as above, but in Simulation Mode.
import ch.aplu.robotsim.*;
public class BorderFollowerS
{
public BorderFollowerS()
{
LegoRobot robot = new LegoRobot();
LightSensor ls = new LightSensor(SensorPort.S1);
robot.addPart(ls);
Gear gear = new Gear();
gear.setSpeed(20);
robot.addPart(gear);
while (true)
{
if (ls.getValue() < 500)
gear.leftArc(0.2);
else
gear.rightArc(0.2);
}
}
public static void main(String[] args)
{
new BorderFollowerS();
}
}
Execute the program locally using WebStart.
|